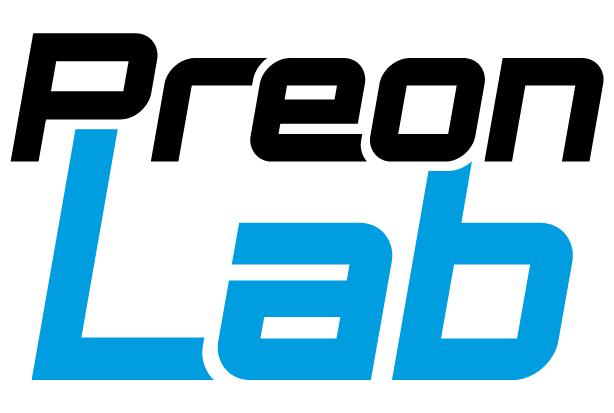
preonpy API 2.2¶
Examples¶
The following example shows how create a new scene. Here we create some objects, set keyframes and save the scene.
import preonpy
s = preonpy.Scene()
s.create_object("Preon Solver")
source = s.create_object("Square source")
box = s.create_object("Box")
position_keyframes = [(0, 0, "Linear"), (1, -1, "Linear"), (2, 0, "Linear"), (3, 2.5, "Linear")]
source.set_keyframes("position x", position_keyframes)
box.set_keyframes("position x", position_keyframes)
s.save("~/moving_box.prscene")
We can also manipulate existing scenes. The next example opens the previous scene again, changes some properties, like the solver spacing and runs a simulation.
s = preonpy.Scene("~/moving_box.prscene")
solver = s.find_object("PreonSolver_1")
solver["spacing"] = 0.1
s.simulate(0, 30)
s.save()
The scene class¶
-
class
preonpy.
Scene
(scene_path=None)¶ Opening new or existing scenes is done by creating Scene objects. If a new scene is created, the previous one gets invalid. Only one scene can be valid at a time. Operations on an invalid scene or its objects result in an exception of type preonpy.InvalidObjectException. The same holds for otherwise deleted objects. Whether a scene (or object) is valid or not can be determined by the is_valid() method.
If scene_path is passed, an existing scene is loaded from the specified path. If omitted, a new scene is created.
If the scene could not be loaded, SceneLoadFailed is raised.
-
create_object
(typename, name=None)¶ Creates a new object of the given type.
Parameters: - typename – The type is specified as a string. You can find the list of supported type names by opening PreonLab and inspecting the Add menu. The type names in preonpy always match the names found in this menu (for instance “Preon Solver” or “Cuboid”).
- name – The object name is an optional parameter. If no name is given, a name is generated.
Returns: The created object.
Raises: ObjectCreateError – If object could not be created.
-
delete_object
(object)¶ Deletes the given object.
Parameters: object – Object to be deleted.
Raises: - ObjectInvalid – If the given object or the scene is invalid.
- ObjectDeleteError – If the object could not be deleted (e.g. because it is a static object).
-
elapsed_time
¶ Returns the current elapsed time of the simulation.
-
find_object
(name)¶ Retrieves an object with the given name or none if the object could not be found.
Parameters: name – The object name. Returns: The object or None if no object with the given name could be found.
-
load_frame
(frame)¶ Loads the given simulation frame.
-
object_names
¶ A list of all object names in the scene.
-
path
¶ Path of the scene file.
-
post_process
(start, stop)¶ Runs post-processing on an existing simulation from the given start to the given end frame.
-
reset_simulationdata
()¶ Removes all previously generated simulation data and sets the current time to zero.
-
save
(scene_path=None, as_portable=False, compression=None, subsample=1)¶ Saves the scene.
Parameters: - scene_path – If scene_path is given, the scene will be saved to the given location. Otherwise, the loaded scene file will be overwritten.
- as_portable – If as_portable is True, dependent resources will be copied into the scene directory if necessary, so that it is simple to transfer the scene and all resources to another place.
- compression – Compression of fluid data can be enabled by setting compression to a non-zero allowed compression error which is a percentage of the used fluid spacing.
- subsample – Setting subsample to a integer value greater than 1 reduces the number of written frames. For instance, pass 2 in order to half the frame rate, discarding every second frame.
Raises: - ValueError – If the scene_path was not valid.
- SceneSaveFailed – If the scene could not be saved.
-
simulate
(start, stop)¶ Runs the simulation from the given start frame to the given end frame.
-
simulate_step
()¶ Runs a single simulation step from the current state. This may be less than a frame.
Usage example:
>>> with scene.simulate_step() as step: >>> for _ in range(100): >>> step()
-
working_dir
¶ Working directory of the scene.
-
The object class¶
-
class
preonpy.
Object
¶ Base class for all objects in the scene.
Object property access is performed using the [] operator. For instance, you can access the position of an object using object[“position”]. The available property names match the property names displayed in the property editor of PreonLab. You can also view a list of available properties by calling object.property_names.
-
__getitem__
(property_name)¶ Returns the value of the property with the given name.
Parameters: property_name – Name of the property, which should be returned. Raises: PropertyNotFound – If the property name could no be found. Example:
>>> property_value = object["property_name"]
-
__setitem__
(property_name, new_value)¶ Sets the value of the property with the given name.
Parameters: - property_name – Name of the property, which should be set.
- new_value – Value to be set.
Raises: PropertyNotFound – If the property name could no be found.
Example:
>>> object["property_name"] = new_value
-
get_connected_objects
(slot_name, outgoing)¶ Retrieves a list of connected objects for the given slot.
Parameters: - slot_name – The name of the connection slot. The name is the same that is displayed in the PreonLab connection editor, for instance “Transform”.
- outgoing – Specifies whether outgoing or incoming connections should be returned.
Returns: List of objects.
Raises: ConnectionError – If an error occurs.
-
get_keyframes
(sequence_name)¶ Retrieves the keyframes for the given sequence.
Parameters: sequence_name – The name of the keyframe sequence, for instance “position x”. Returns: A list of triples, in which the first triple value indicates the time, the second the value and the third the interpolation string type for the following segment. Raises: KeyframeError – If an error occurs.
-
get_property_enum_values
(name)¶ Returns valid enum values.
Parameters: name – Name of the property. Must be an enum property.
Returns: The possible values for the given property.
Raises: - PropertyError – If the property is no enum.
- PropertyNotFound – If the property name could no be found.
-
get_property_help
(name)¶ Returns property description.
Parameters: name – Name of the property. Returns: A short description of the given property. Raises: PropertyNotFound – If the property name could no be found.
-
get_property_type
(name)¶ Returns property type.
Parameters: name – Name of the property. Returns: The type of the property with the given name. Raises: PropertyNotFound – If the property name could no be found.
-
get_statistic
(name, time)¶ Returns the value of the statistic identified by name at the given time.
Parameters: - name – The name of the statistic which must be one of the statistics that is displayed in PreonLab in the on-screen display (for instance “flow rate” for sensor planes).
- time – The time at which the statistic is queried.
Returns: The value of the statistic identified by name at the given time.
Raises: StatisticNotAvailable – If the statistic does not exist anymore.
-
get_statistic_avg
(name, start_time, end_time)¶ Returns the average of the statistic values identified by name in the given time range.
Parameters: - name – The name of the statistic which must be one of the statistics that is displayed in PreonLab in the on-screen display (for instance “flow rate” for sensor planes).
- start_time – The start time for statistic averaging.
- end_time – The end time for statistic averaging.
Returns: The value of the statistic identified by name at the given time.
Raises: - StatisticNotAvailable – If the statistic does not exist anymore.
- ValueError – end_time and start_time are invalid.
-
get_statistic_max
(name)¶ Returns the maximum value that the given statistic has over time.
Parameters: name – The name of the statistic which must be one of the statistics that is displayed in PreonLab in the on-screen display (for instance “flow rate” for sensor planes). Returns: The maximum value over time of the statistic identified by name. Raises: StatisticNotAvailable – If the statistic does not exist anymore.
-
get_statistic_min
(name)¶ Returns the minimum value that the given statistic has over time.
Parameters: name – The name of the statistic which must be one of the statistics that is displayed in PreonLab in the on-screen display (for instance “flow rate” for sensor planes). Returns: The minimum value over time of the statistic identified by name. Raises: StatisticNotAvailable – If the statistic does not exist anymore.
-
is_valid
()¶ Returns True if the object is valid.
-
property_names
¶ Returns a list of property names. Properties can be accessed via the index operator.
-
set_keyframes
(sequence_name, keyframes)¶ Sets the keyframes for the given sequence name.
Parameters: - sequence_name – The name of the keyframe sequence, for instance “position x”.
- keyframes – A list of triples, in which the first triple value indicates the time, the second the value and the third the interpolation string type for the following segment (pass “Linear” for linear interpolation or “Step” for step interpolation).
Raises: KeyframeError – If an error occurs.
-
statistic_names
¶ A list with names of the available statistics. Note that simulation data has to be available.
Returns: A list of the object’s statistic names.
-
write_statistics_to_csv
(file_path)¶ Write statistics data as CSV to the given file.
-
Object specific functionality¶
Solid objects¶
-
class
preonpy.
Rigid
¶ -
raycast
(ray_origin, ray_dir)¶ Casts a ray from the given origin into the given direction and returns the distance to the closest hit. Returns -1 if no hit was found. This function may be computationally very expensive depending on the amount of triangles in the mesh.
Parameters: - ray_origin – The origin position of the ray.
- ray_dir – The direction of the ray.
-
Sensor plane, Air Flow Visualizer, Height field and Velocity projection field¶
-
class
preonpy.
FloatFieldWrapper
¶ Base class for sensor planes, air flow visualizers, height fields and velocity projection fields.
-
get_projected_value
(position, default)¶ Projects position on the 2D field and returns the value stored at that position. If the projected point is outside the 2D field, the default value is returned.
-
-
class
preonpy.
SensorPlane
¶ -
export_sample_data_to_csv
(start, stop, local_coords)¶ - Exports the sample data of a SensorPlane as CSV file(s). The frame number is used for naming the file(s).
- The exported files are stored in the respective subfolder of the folder ‘SensorData’ in the scene directory.
Parameters: - start – First frame.
- stop – Last frame. Can be equal to start.
- local_coords – If True, the data is saved in the local space of the plane, else the global scene space is used.
-
-
class
preonpy.
ForceSensor
¶ -
export_sample_data_to_csv
(start, stop, local_coords, save_pressure)¶ - Exports the sample data of a ForceSensor as CSV file(s). The frame number is used for naming the file(s).
- The exported files are stored in the respective subfolder of the folder ‘SensorData’ in the scene directory.
Parameters: - start – First frame.
- stop – Last frame. Can be equal to start.
- local_coords – If True, the data is saved in the local space of the object, else the global scene space is used.
- save_pressure – If True, the data is saved as pressure in Pascal.
-
Preon Renderer¶
-
class
preonpy.
PreonRenderer
¶ -
render
(start, stop, cam_objects=None)¶ Render the frames in the given range for one or multiple cameras.
Parameters: - start – First frame.
- stop – Last frame. Can be equal to start.
- cam_objects – Camera object or list of camera objects. Renderer uses connected camera, if omitted.
-
Object connections¶
-
preonpy.
connect_objects
(obj1, obj2, slot_name)¶ Creates a connection from obj1 to obj2 for the given connection slot name.
Parameters: slot_name – The name of the connection slot. The name is the same that is displayed in the PreonLab connection editor, for instance “Transform”. Raises: ConnectionError – If the objects could not be connected.
-
preonpy.
disconnect_objects
(obj1, obj2, slot_name)¶ Removes the connection from obj1 to obj2 for the given connection slot name.
Parameters: slot_name – The name of the connection slot. The name is the same that is displayed in the PreonLab connection editor, for instance “Transform”. Raises: ConnectionError – If the objects could not be connected.
Utility classes¶
-
class
preonpy.
Vec3
(x, y, z)¶ -
x
¶ Alias for field number 0
-
y
¶ Alias for field number 1
-
z
¶ Alias for field number 2
-
Miscellaneous¶
-
preonpy.
get_license_path
()¶ Returns the path on which the license file is expected.
-
preonpy.
get_logfile_path
()¶ Returns the path of the log file.
-
preonpy.
get_version
()¶ Returns the version string of PreonLab.
-
preonpy.
show_progressbar
¶ Configures if a progressbar is shown for some long running tasks like simulate or post_process. This optional feature requires the progressbar2 package to be installed. The default value is True if the package is installed and False otherwise.
-
preonpy.
scene
¶ Holds the current scene or None if no scene is loaded. Only meant for read access.
Exceptions¶
-
class
preonpy.
PreonpyException
¶ Base class for all Exceptions preonpy raises.
-
class
preonpy.
ObjectInvalid
¶ Raised whenever an invalid object (or scene) was accessed.
-
class
preonpy.
PropertyError
¶ Base type for exceptions related to property access.
-
class
preonpy.
PropertyReadTypeError
(key)¶ Raised on property read access if the property type is not readable.
-
class
preonpy.
PropertyWriteTypeError
(key)¶ Raised on property write access if the property type is not writeable.
-
class
preonpy.
PropertyReadonlyError
(key)¶ Raised on property write access if the property is readonly.
-
class
preonpy.
PropertyWriteError
(key, item_type, value, index, metautil)¶ Raised if a property write failed, because of the given value was invalid.
-
class
preonpy.
KeyframeError
¶ Raised one errors related to keyframe access.
-
class
preonpy.
ConnectionError
¶ Raised one errors related to object connections.
-
class
preonpy.
StatisticNotAvailable
(name)¶ Raised if statistic is not available.
-
class
preonpy.
SceneLoadFailed
(scene_path)¶ Raised if scene load failed.
-
class
preonpy.
SceneSaveFailed
(scene_path)¶ Raised if scene save failed.
-
class
preonpy.
ObjectCreateError
(typename)¶ Raised if object could not be created.
-
class
preonpy.
ObjectDeleteError
(objname)¶ Raised if object could not be deleted.